해당 자료는 w3schools을 참조하여 작성했습니다.
https://www.w3schools.com/java/java_modifiers.asp
Java Modifiers
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.
www.w3schools.com
Modifier의 종류
Modifier는 다음과 같이 두가지 그룹으로 나눌 수 있습니다:
- Access Modifiers - access level을 제어합니다.
- Non-Access Modifiers - access level을 제어하지는 않지만, 다른 기능을 제공합니다.
Access Modifier
Class의 경우 public
또는 defalut을 사용할 수 있습니다:
Modifier | 설명 | 예제 코드 |
public |
이 class는 다른 class에서 접근할 수 있습니다. | Try it » |
default | 이 class는 동일한 package의 class에서만 접근할 수 있습니다. modifier를 지정하지 않을 때 기본값으로 사용됩니다. | Try it » |
Attributes, methods와 constructors의 경우 다음 중 하나를 사용할 수 있습니다:
Modifier | 설명 | 예제 코드 |
public |
이 code는 다른 class에서 접근할 수 있습니다. | Try it » |
private |
이 code는 선언된 class 내에서만 접근할 수 있습니다. | Try it » |
default | 이 code는 동일한 package에서만 접근할 수 있습니다. modifier를 지정하지 않을 때 기본값으로 사용됩니다. | Try it » |
protected |
이 code는 동일한 package 및 하위 클래스에서 접근할 수 있습니다. | Try it » |
Non-Access Modifier
Class의 경우 final
또는 abstract
를 사용할 수 있습니다:
Modifier | 설명 | 예제 코드 |
final |
이 class는 다른 class에서 상속할 수 없습니다. | Try it » |
abstract |
이 class는 object를 만들 수 없습니다. (abstract class에 접근하려면 다른 class에서 상속해야 합니다.) | Try it » |
Attributes와 method에서는 다음 중 하나를 사용할 수 있습니다:
Modifier | 설명 | 예제 코드 |
final |
Attributes와 method는 재정의하거나 수정할 수 없습니다. | Try it » |
static |
Attributes와 method는 object가 아닌 class에 속합니다. | Try it » |
abstract |
abstract class안의 method에서만 사용할 수 있습니다. 상속받는 하위 class에서 method의 정의를 내립니다. | Try it » |
transient |
Attributes나 methodd가 포함된 object를 직렬화(serializing)할 때, 해당 attributes나 method를 건너뜁니다.(직렬화 대상에서 제외) | |
synchronized |
method는 한 번에 하나의 thread만 접근할 수 있습니다. (다른 접근은 허용하지 않습니다.) | |
volatile |
Attributes의 값은 thread-locally에 캐시되지 않으며 항상 "기본 메모리"에서 읽힙니다. |
transient 예제 코드
// A serialization demo.
// This program uses try-with-resources. It requires JDK 7 or later.
import java.io.*;
public class SerializationDemo{
public static void main(String args[]){
// Object serialization
try ( ObjectOutputStream objOStrm =
new ObjectOutputStream(new FileOutputStream("serial")) )
{
MyClass object1 = new MyClass("Serializing and Reverse", 10, 3.1e5);
System.out.println("object1: " + object1);
objOStrm.writeObject(object1);
}
catch(IOException e) {
System.out.println("Exception during serialization: " + e);
}
// Object deserialization
try ( ObjectInputStream objIStrm =
new ObjectInputStream(new FileInputStream("serial")) )
{
MyClass object2 = (MyClass)objIStrm.readObject();
System.out.println("object2: " + object2);
}
catch(Exception e) {
System.out.println("Exception during deserialization: " + e);
}
}
}
class MyClass implements Serializable{
String s;
transient int i;
double d;
public MyClass(String s, int i, double d){
this.s = s;
this.i = i;
this.d = d;
}
public String toString(){
return "s=" + s + "; i=" + i + "; d=" + d;
}
}
Output:
object1: s=Serializing and Reverse; i=10; d=310000.0
object2: s=Serializing and Reverse; i=0; d=310000.0
transient가 없었더라면 i 값은 다른 값들과 마찬가지로 ObjectIOStream을 통해서 전달됩니다. 하지만 transient modifier가 붙어있기 때문에 직렬화 대상에서 제외되어 초기값인 0을 출력하는 것입니다.
volatile 추가 설명
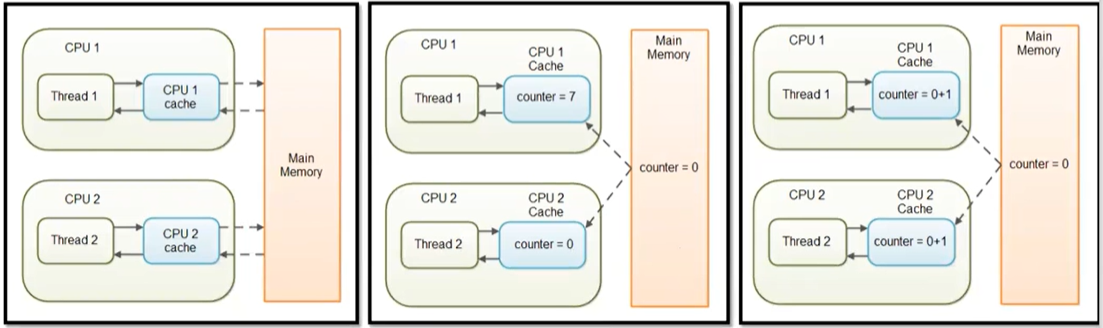
multi-core system을 갖춘 환경이라고 해보자. 각 CPU(core)에서는 CPU만의 cache가 존재합니다. 일반적인 상황의 경우 Task를 수행하는 동안 성능 향상을 위해 main memory에서 읽은 변수 counter를 각 CPU의 cache에 저장합니다. 각각 변수의 값을 읽어올 때 동시 사용 중에는 cache에 저장한 값이 다를 수 있기 때문에 변수 값이 일치하지않는 문제가 발생합니다. 하지만 volatile modifier를 사용한 경우, main memory에서 읽어온 값을 memory에 저장하기 때문에 해당 문제를 해결할 수 있습니다.
public class VolatileMain {
private final static int noOfThreads = 3;
public static void main(String[] args) throws InterruptedException {
VolatileData volatileData = new VolatileData();
Thread[] threads = new Thread[noOfThreads];
for (int i = 0; i < noOfThreads; ++i)
threads[i] = new VolatileThread(volatileData);
for (int i = 0; i < noOfThreads; ++i)
threads[i].start();
}
}
public class VolatileData {
private int counter = 0;
// private volatile int counter = 0;
// volatile이 있고 없고의 차이: 변수 값의 업데이트가 cache인지 memory인지 차이
public int getCounter() {
return counter;
}
public void increaseCounter() {
++counter;
}
}
public class VolatileThread extends Thread {
private VolatileData data;
public VolatileThread(VolatileData data) {
this.data = data;
}
@Override
public void run() {
int oldValue = data.getCounter();
System.out.println("[Thread " + Thread.currentThread().getId() + "]: Old value = " + oldValue);
data.increaseCounter();
int newValue = data.getCounter();
System.out.println("[Thread " + Thread.currentThread().getId() + "]: New value = " + newValue);
}
}
해당 자료는 w3schools을 참조하여 작성했습니다.
https://www.w3schools.com/java/java_modifiers.asp
Java Modifiers
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.
www.w3schools.com
Modifier의 종류
Modifier는 다음과 같이 두가지 그룹으로 나눌 수 있습니다:
- Access Modifiers - access level을 제어합니다.
- Non-Access Modifiers - access level을 제어하지는 않지만, 다른 기능을 제공합니다.
Access Modifier
Class의 경우 public
또는 defalut을 사용할 수 있습니다:
Modifier | 설명 | 예제 코드 |
public |
이 class는 다른 class에서 접근할 수 있습니다. | Try it » |
default | 이 class는 동일한 package의 class에서만 접근할 수 있습니다. modifier를 지정하지 않을 때 기본값으로 사용됩니다. | Try it » |
Attributes, methods와 constructors의 경우 다음 중 하나를 사용할 수 있습니다:
Modifier | 설명 | 예제 코드 |
public |
이 code는 다른 class에서 접근할 수 있습니다. | Try it » |
private |
이 code는 선언된 class 내에서만 접근할 수 있습니다. | Try it » |
default | 이 code는 동일한 package에서만 접근할 수 있습니다. modifier를 지정하지 않을 때 기본값으로 사용됩니다. | Try it » |
protected |
이 code는 동일한 package 및 하위 클래스에서 접근할 수 있습니다. | Try it » |
Non-Access Modifier
Class의 경우 final
또는 abstract
를 사용할 수 있습니다:
Modifier | 설명 | 예제 코드 |
final |
이 class는 다른 class에서 상속할 수 없습니다. | Try it » |
abstract |
이 class는 object를 만들 수 없습니다. (abstract class에 접근하려면 다른 class에서 상속해야 합니다.) | Try it » |
Attributes와 method에서는 다음 중 하나를 사용할 수 있습니다:
Modifier | 설명 | 예제 코드 |
final |
Attributes와 method는 재정의하거나 수정할 수 없습니다. | Try it » |
static |
Attributes와 method는 object가 아닌 class에 속합니다. | Try it » |
abstract |
abstract class안의 method에서만 사용할 수 있습니다. 상속받는 하위 class에서 method의 정의를 내립니다. | Try it » |
transient |
Attributes나 methodd가 포함된 object를 직렬화(serializing)할 때, 해당 attributes나 method를 건너뜁니다.(직렬화 대상에서 제외) | |
synchronized |
method는 한 번에 하나의 thread만 접근할 수 있습니다. (다른 접근은 허용하지 않습니다.) | |
volatile |
Attributes의 값은 thread-locally에 캐시되지 않으며 항상 "기본 메모리"에서 읽힙니다. |
transient 예제 코드
// A serialization demo.
// This program uses try-with-resources. It requires JDK 7 or later.
import java.io.*;
public class SerializationDemo{
public static void main(String args[]){
// Object serialization
try ( ObjectOutputStream objOStrm =
new ObjectOutputStream(new FileOutputStream("serial")) )
{
MyClass object1 = new MyClass("Serializing and Reverse", 10, 3.1e5);
System.out.println("object1: " + object1);
objOStrm.writeObject(object1);
}
catch(IOException e) {
System.out.println("Exception during serialization: " + e);
}
// Object deserialization
try ( ObjectInputStream objIStrm =
new ObjectInputStream(new FileInputStream("serial")) )
{
MyClass object2 = (MyClass)objIStrm.readObject();
System.out.println("object2: " + object2);
}
catch(Exception e) {
System.out.println("Exception during deserialization: " + e);
}
}
}
class MyClass implements Serializable{
String s;
transient int i;
double d;
public MyClass(String s, int i, double d){
this.s = s;
this.i = i;
this.d = d;
}
public String toString(){
return "s=" + s + "; i=" + i + "; d=" + d;
}
}
Output:
object1: s=Serializing and Reverse; i=10; d=310000.0
object2: s=Serializing and Reverse; i=0; d=310000.0
transient가 없었더라면 i 값은 다른 값들과 마찬가지로 ObjectIOStream을 통해서 전달됩니다. 하지만 transient modifier가 붙어있기 때문에 직렬화 대상에서 제외되어 초기값인 0을 출력하는 것입니다.
volatile 추가 설명
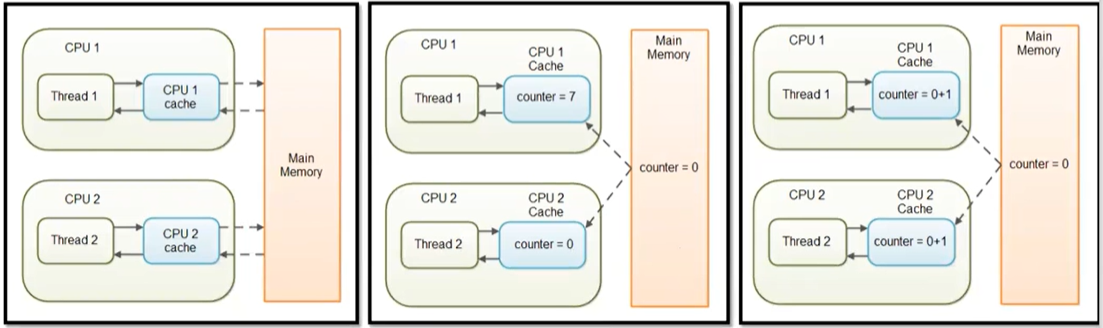
multi-core system을 갖춘 환경이라고 해보자. 각 CPU(core)에서는 CPU만의 cache가 존재합니다. 일반적인 상황의 경우 Task를 수행하는 동안 성능 향상을 위해 main memory에서 읽은 변수 counter를 각 CPU의 cache에 저장합니다. 각각 변수의 값을 읽어올 때 동시 사용 중에는 cache에 저장한 값이 다를 수 있기 때문에 변수 값이 일치하지않는 문제가 발생합니다. 하지만 volatile modifier를 사용한 경우, main memory에서 읽어온 값을 memory에 저장하기 때문에 해당 문제를 해결할 수 있습니다.
public class VolatileMain {
private final static int noOfThreads = 3;
public static void main(String[] args) throws InterruptedException {
VolatileData volatileData = new VolatileData();
Thread[] threads = new Thread[noOfThreads];
for (int i = 0; i < noOfThreads; ++i)
threads[i] = new VolatileThread(volatileData);
for (int i = 0; i < noOfThreads; ++i)
threads[i].start();
}
}
public class VolatileData {
private int counter = 0;
// private volatile int counter = 0;
// volatile이 있고 없고의 차이: 변수 값의 업데이트가 cache인지 memory인지 차이
public int getCounter() {
return counter;
}
public void increaseCounter() {
++counter;
}
}
public class VolatileThread extends Thread {
private VolatileData data;
public VolatileThread(VolatileData data) {
this.data = data;
}
@Override
public void run() {
int oldValue = data.getCounter();
System.out.println("[Thread " + Thread.currentThread().getId() + "]: Old value = " + oldValue);
data.increaseCounter();
int newValue = data.getCounter();
System.out.println("[Thread " + Thread.currentThread().getId() + "]: New value = " + newValue);
}
}